Python Pathlib
파일 경로를 다루는 것은 프로그래밍에서 기본적이면서도 중요한 작업입니다. Python에서는 전통적으로 os.path
모듈을 사용해 왔지만, Python 3.4부터 도입된 pathlib
모듈은 객체 지향적이고 직관적인 방식으로 파일 시스템 경로를 다룰 수 있게 해줍니다. 이 글에서는 pathlib
의 설치부터 주요 기능, 실용적인 예제까지 살펴보겠습니다.
Pathlib 설치 방법
좋은 소식은 pathlib
이 Python 3.4 이상 버전에서는 표준 라이브러리에 포함되어 있다는 것입니다. 따라서 별도의 설치 없이 바로 사용할 수 있습니다.
# 간단히 import 하여 사용
import pathlib
# 또는 주로 사용되는 Path 클래스만 import
from pathlib import Path
Python 3.4 미만 버전을 사용하는 경우에는 pip를 통해 설치할 수 있습니다:
pip install pathlib
Pathlib의 주요 클래스와 함수
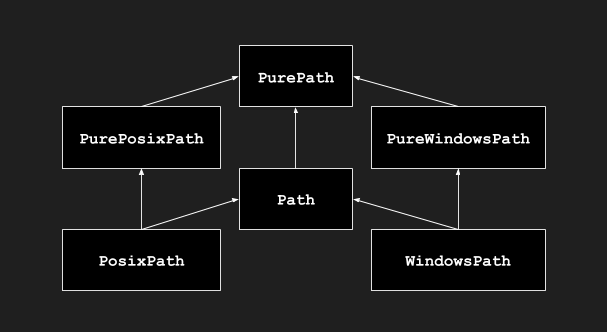
출처:https://docs.python.org/ko/3.13/library/pathlib.html#concrete-paths
주요 클래스
- Path: 운영 체제에 따라 적절한 경로 클래스를 반환하는 일반적인 클래스
- PurePath: 순수 경로 조작을 위한 기본 클래스 (파일 시스템 액세스 없음)
- PurePosixPath: POSIX 스타일 경로를 위한 클래스
- PureWindowsPath: Windows 스타일 경로를 위한 클래스
- PosixPath: POSIX 시스템에서 파일 시스템 액세스 기능이 있는 클래스
- WindowsPath: Windows 시스템에서 파일 시스템 액세스 기능이 있는 클래스
주요 메서드와 속성
- 경로 정보 속성:
name
,stem
,suffix
,parent
,parents
- 경로 조작:
joinpath()
,/
연산자,with_name()
,with_suffix()
- 파일 시스템 액세스:
exists()
,is_file()
,is_dir()
,stat()
- 파일 작업:
open()
,read_text()
,write_text()
,read_bytes()
,write_bytes()
- 디렉토리 작업:
mkdir()
,rmdir()
,iterdir()
,glob()
,rglob()
Pathlib 사용 방법
기본 경로 생성
from pathlib import Path
# 현재 작업 디렉토리
current_path = Path.cwd()
print(current_path)
# 홈 디렉토리
home_path = Path.home()
print(home_path)
# 상대 경로
relative_path = Path('docs/report.txt')
print(relative_path)
# 절대 경로
absolute_path = Path('/usr/local/bin')
print(absolute_path)
경로 결합
# 방법 1: / 연산자 사용 (권장)
data_file = Path('data') / 'input.csv'
print(data_file)
# 방법 2: joinpath 메서드 사용
config_file = Path('config').joinpath('settings.json')
print(config_file)
# 여러 경로 결합
log_file = Path('var') / 'log' / 'app' / 'error.log'
print(log_file)
경로 정보 얻기
file_path = Path('/home/user/documents/report.pdf')
# 파일 이름
print(file_path.name) # 'report.pdf'
# 파일 이름(확장자 제외)
print(file_path.stem) # 'report'
# 파일 확장자
print(file_path.suffix) # '.pdf'
# 부모 디렉토리
print(file_path.parent) # PosixPath('/home/user/documents')
# 모든 부모 디렉토리 리스트
for parent in file_path.parents:
print(parent)
실용적인 사용 예제
예제 1: 특정 확장자 파일 찾기
from pathlib import Path
# 현재 디렉토리의 모든 Python 파일 찾기
python_files = list(Path.cwd().glob('*.py'))
for file in python_files:
print(file)
# 하위 디렉토리를 포함한 모든 Python 파일 찾기
all_python_files = list(Path.cwd().rglob('*.py'))
for file in all_python_files:
print(file)
예제 2: 파일 읽기/쓰기
from pathlib import Path
# 텍스트 파일 쓰기
file_path = Path('example.txt')
file_path.write_text('Hello, Pathlib!')
# 텍스트 파일 읽기
content = file_path.read_text()
print(content)
# 바이너리 파일 쓰기
binary_path = Path('binary_file.bin')
binary_path.write_bytes(b'\x00\x01\x02\x03')
# 바이너리 파일 읽기
binary_data = binary_path.read_bytes()
print(binary_data)
예제 3: 디렉토리 작업
from pathlib import Path
# 디렉토리 생성
new_dir = Path('new_directory')
new_dir.mkdir(exist_ok=True)
# 중첩 디렉토리 생성
nested_dir = Path('parent/child/grandchild')
nested_dir.mkdir(parents=True, exist_ok=True)
# 디렉토리 내용 나열
for item in Path('parent').iterdir():
if item.is_file():
print(f'파일: {item}')
elif item.is_dir():
print(f'디렉토리: {item}')
예제 4: 파일 이동 및 이름 변경
from pathlib import Path
import shutil
# 파일 이동
source = Path('source.txt')
source.write_text('This is a test file')
destination = Path('destination') / 'moved.txt'
destination.parent.mkdir(exist_ok=True)
# pathlib에는 직접적인 이동 메서드가 없어 shutil 사용
shutil.move(str(source), str(destination))
# 파일 이름 변경
old_name = Path('old_name.txt')
old_name.write_text('Rename me')
new_name = old_name.with_name('new_name.txt')
old_name.rename(new_name)
# 확장자 변경
text_file = Path('document.txt')
text_file.write_text('Change my extension')
markdown_file = text_file.with_suffix('.md')
text_file.rename(markdown_file)
Pathlib의 유용한 팁
1. os.path와의 호환성
기존 코드와의 호환성을 위해 Path 객체를 문자열로 변환해야 할 때가 있습니다:
import os
from pathlib import Path
path = Path('some_file.txt')
# Path 객체를 문자열로 변환
path_str = str(path)
os.path.exists(path_str) # os.path 함수와 함께 사용
Python 3.6부터는 많은 os 함수들이 Path 객체를 직접 받을 수 있습니다:
import os
from pathlib import Path
path = Path('some_file.txt')
os.path.exists(path) # 직접 Path 객체 전달 가능
2. 홈 디렉토리 확장
from pathlib import Path
# 틸드(~)를 홈 디렉토리로 확장
home_config = Path('~/.config').expanduser()
print(home_config)
3. 상대 경로와 절대 경로 변환
from pathlib import Path
relative_path = Path('docs/report.txt')
absolute_path = relative_path.absolute()
print(absolute_path)
# 현재 작업 디렉토리에 대한 상대 경로 얻기
some_path = Path('/usr/local/bin/python')
relative_to_cwd = some_path.relative_to(Path.cwd())
print(relative_to_cwd)
4. 파일 시스템 상태 확인
from pathlib import Path
import time
file_path = Path('example.txt')
# 파일 존재 여부 확인
if file_path.exists():
# 파일 크기
print(f'파일 크기: {file_path.stat().st_size} 바이트')
# 마지막 수정 시간
mtime = file_path.stat().st_mtime
print(f'마지막 수정: {time.ctime(mtime)}')
# 파일 권한
print(f'파일 권한: {oct(file_path.stat().st_mode)[-3:]}')
결론
pathlib
모듈은 Python에서 파일 시스템 경로를 다루는 방식을 현대적이고 직관적으로 개선했습니다. 객체 지향적 접근 방식은 코드의 가독성을 높이고, 다양한 내장 메서드는 파일 시스템 작업을 간소화합니다. 특히 경로 결합을 위한 /
연산자 사용, 파일 내용 읽기/쓰기를 위한 간결한 메서드, 그리고 강력한 패턴 매칭 기능은 pathlib
의 큰 장점입니다.
기존의 os.path
모듈에 익숙한 개발자들도 pathlib
으로 전환하면 더 깔끔하고 유지보수하기 쉬운 코드를 작성할 수 있을 것입니다. Python으로 파일 시스템을 다루는 모든 개발자에게 pathlib
모듈의 사용을 적극 권장합니다.
답글 남기기